Implement a Rectangle
class with the following properties.
A default Rectangle
object is specified in the constructor with the x
, y
, width
and height
set to 0.
A Rectangle
object is specified in the constructor with the left and right edges of the rectangle at x
and x + width
. The top and bottom edges are at y
and y + height
.
A Rectangle
object is specified that is a copy of an existing Rectangle
.
Methods getXPos
, getYPos
, getWidth
, and getHeight
, return the x
, y
, height
and width
of the Rectangle
respectively.
A method, getDirection
, returns the current orientation of the DrawingTool
.
Methods setXPos
, setYPos
, setWidth
, and setHeight
, sets the x
, y
, height
and width
of the Rectangle
respectively to the value of each methods double
parameter.
A method setDirection
, sets the current orientation of the DrawingTool
.
A method getPerimeter
calculates and returns the perimeter of the Rectangle
.
A method getArea
calculates and returns the area of the Rectangle
.
A method draw
displays a new instance of a Rectangle
object.
A method drawString
displays String at the specified x and y coordinates of the drawing area.
The methods draw
, drawString
, and setDirection
make use of existing DrawingTool
methods. Refer to
DrawingTool,
documentation for details on the DrawingTool
methods.
Write a testing class with a main method that constructs a Rectangle
, rectA
, and calls setDirection
, setWidth
, and draw
for each Rectangle
created. It is recommended that the changes in orientation and width of each successive rectangle in the spiral be calculated using the getDirection
and getWidth
methods. For example, if the increment for each turn is given by turnInc
and the decrease in size of rectangle is given by widthDec
, then successive calls to the following:
rectA.setDirection(rectA.getDirection() - turnInc);
rectA.setWidth(rectA.getWidth() - widthDec);
rectA.draw();
would draw each "spoke" of the rectangular spiral:
Construct another Rectangle
, rectB
, that is a copy of the orginal rectA
. Draw the rectangle in the upper left corner of drawing area. Label the rectangle with its width, height, perimeter and area.
The resulting image would be similar to the one shown below:
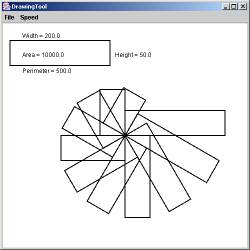
Submit the project including the source code and the run
output image. It is recommended that the Rectangle
class and the testing class be
in separate source files (Rectangle.java and
RectangleTester.java).