Methods for the ListNode
class will consist of those for creating, accessing, and modifying nodes.
An implementation of a generic ListNode
class are shown
below.
/**
* Implementation of a node of a singly linked list.
*
* Adapted from the College Board's AP Computer Science AB: Implementation
* Classes and Interfaces.
*/
public class ListNode<E>
{
private E value;
private ListNode next;
// Constructor
public ListNode( E initValue, ListNode initNext )
{
value = initValue;
next = initNext;
}
// Returns value of list node.
public E getValue()
{
return value;
}
// Returns the next field of ListNode.
public ListNode getNext()
{
return next;
}
// Sets the value of the list node to theNewValue
public void setValue( E theNewValue )
{
value = theNewValue;
}
// Sets the list node's next field to a new reference.
public void setNext( ListNode theNewNext )
{
next = theNewNext;
}
}
-
The constructor for the ListNode
class is responsible for creating a node and initializing the two instance variables of a new node.
// postcondition: constructs a new element with object initValue,
//
followed by next element
public ListNode( E initValue, ListNode initNext)
{
value = initValue;
next = initNext;
}
-
Here is an example of code to create the first node of a linked list.
ListNode<Integer> first;
first = new ListNode(new Integer(23), null);
After execution of the two statements, first
refers to the header node of a small linked list that contains just one node with the Integer 23
.
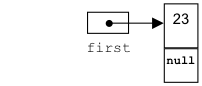
-
Getting and setting the data and link of the node are accomplished with getter and setter methods as follows.
// postcondition: returns value associated with this element
public E getValue()
{
return value;
}
// postcondition: returns reference to next value in list
public ListNode getNext()
{
return next;
}
public void setValue( E theNewValue )
{
value = theNewValue;
}
// postcondition: sets reference to new next value
public void setNext(ListNode theNewNext)
{
next = theNewNext;
}
- The following segment of code using
ListNode
will illustrate the syntax of accessing the data members of a ListNode
.
ListNode<Integer> list;
list = new ListNode(new Integer(13), null);
System.out.println("The node contains: " +
list.getValue());
list.setValue(new Integer(17));
System.out.println("The node contains: " + list.getValue());
Run Output:
The node contains: 13
The node contains: 17