To delete from a singly or a doubly linked list, you need to consider
the same cases as you do when you insert a node into a linked list. Is the
list empty? Is the node that is to be deleted the first node of the list?
is it in the middle? Is it the last node? Is the node to be deleted even
in the list? If the node is not in the list, what should happen? If there
is ore than one node with the same value as the node to be deleted, should
only the first occurrence be deleted or all nodes with that value?
-
To delete from the middle of a doubly linked list, you must adjust two
references.
DLListNode hold = temp.getPrevious();
hold.setNext( temp.getNext() );
DLListNode hold2 = temp.getNext();
hold2.setPrevious( temp.getPrevious() );
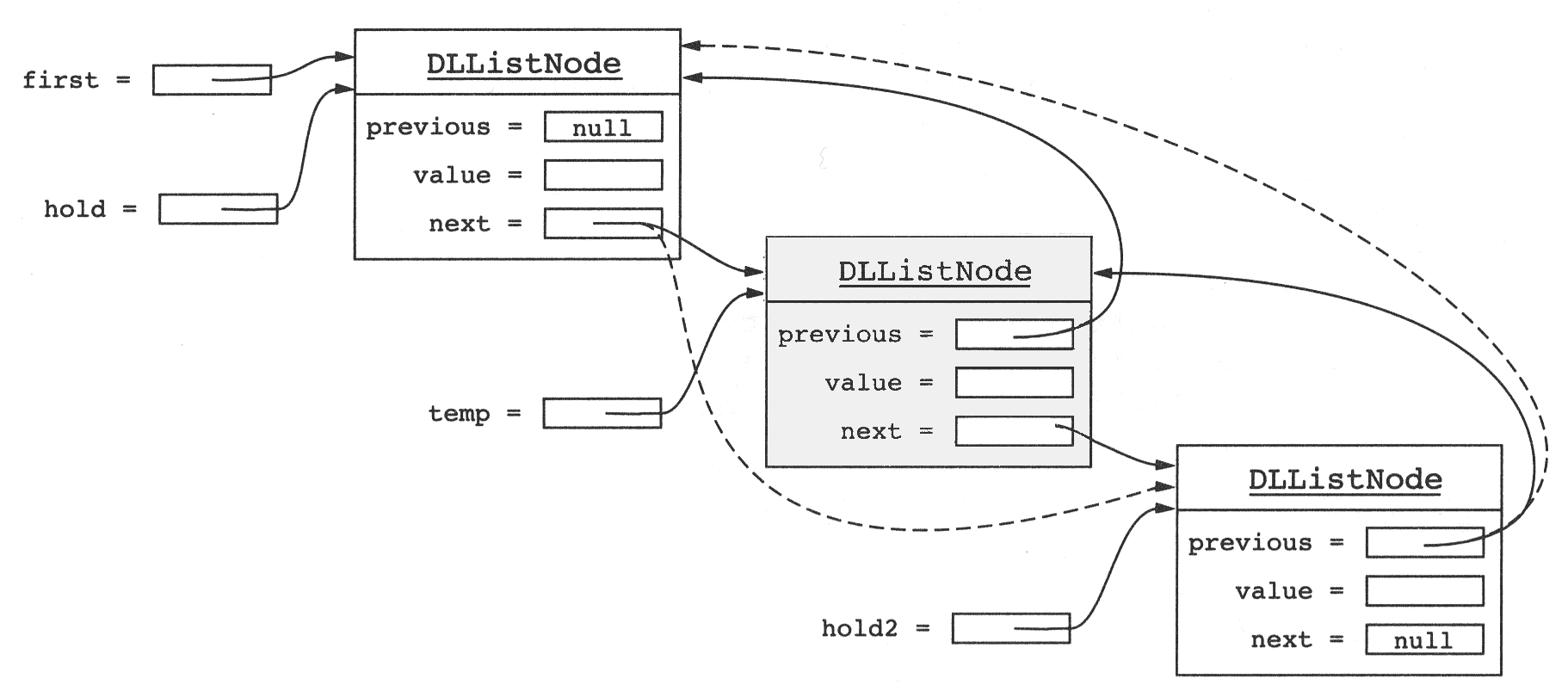
Figure 29-4
It is a good exercise to draw the nodes of a doubly linked list and write the code necessary to delete the first and last nodes of the list.
The Java LinkedList
class is implemented using a doubly linked list
with references to the first node and to the last node. Operations that index into the list (accessing the
ith node) will traverse the list from the beginning or the end, whichever is closer to the specified index
(i)